Welcome
$$ \text{Vitamin C Programming Supplement} $$
Welcome to Vitamin C Programming Supplement. It is a guide like book for programming beginners focusing on the C Programming Language. A lot of resources are available online, but we're here to make a compilation (gather necessary resources here) as well as exercises to enhance your programming skills.
We have tried to make this content easy as possible from a Bangladeshi point of view and written exams. Furthermore, we will be sharing our notes on Programming fundamentals and the C programming language.
It takes a lot of time to master a programming language. You need to put in practice and dedication to achieve proficiency. You can't just read books or watch tutorials; you have to write code yourself and try new things (explore!).
This book is prepared as a supplement to your mainstream learning resource (like YouTube playlists or any books). After completing topics from your main learning source, you can find additional information/knowledge and some practice problems here. Do not take this book as your main book to learn as we have skipped many essential topics believing that you will learn them in your class or YouTube lecture or from books.
Acknowledgement
"আমরা একাত্তর দেখিনি, কিন্তু চব্বিশ দেখেছি" (Bangla Phonetic:"Amra Ekattor Dekhini, Kintu Cobbish Dekhechi") "We haven't seen seventy-one, but have seen twenty-four"
We salute our heroes of 52's Language Movement, 71's Freedom Fighters, Honoured Leaders, 24's fearless activists, who fought bravely against autocratic government, and the martyrs of July Massacre.
Also, we extend our respect to our Structured Programming Course Coordinator for his sincere efforts in guiding us through this course. We dream a beautiful and better world too...
Start Here
Start with C (NOT PYTHON)
Why to learn such old fashioned language like C ?
- It is considered as the mother or backbone of all programming language
- If you have have a good understanding of solving problem with C to learn other programming language like C++, Java, JavaScript, Kotlin (Kotlin needs knowledge of JAVA), Rust, will be easy-peasy !
- Harvard Uni, BUET, almost every university starts Programming courses with C Programming language.
- Linux, Windows Kernel is written in C.
- Good to know: You have direct access to memory that most other languages doesn't. C is like a basic building blocks of everything. You can do everything in C.
- Since C doesn't have enriched library, you need to implement your bigger ideas manually thus having a good understanding over those concepts (like Sorting, Stack, Queue, etc.)
- Python lovers don't know what is happening inside. You can't call yourself a computer engineer if you don't know the mechanism. Let the python lovers and web developers make noise. Don't listen to them. Even, Python and Java BackEnd is written in C. Now, lets start Vitamin C.
Some of the Notes on C are given below: https://github.com/KaziRifatMorshed/WelcomeToProgramming
1. Editor and Compiler Setup
Considering you as newbies we are only going to suggest 2 editors (each) here. If you have any problems installing them then contact us. Until you've got some hands on experience it is better to stick with the first options in both cases.
i. Offline
We prefer to use VS Code and MSYS2 compiler.
Name | Link | Installation | Comment |
---|---|---|---|
Codeblocks | Download | How to setup | Codeblocks is one of the old but featureful IDEs still regarded as good |
Visual Studio Code | Download | How to setup | How to configure VS Code |
ii. Online
Name | Link | Comments |
---|---|---|
Programiz | Let's Code | |
OnlineGDB | Let's Code |
Pros of Online IDE
- Easiest to use
- Clean and Beginner friendly User Interface
Cons of Online IDE
- Need active internet connection
- Can perform basic codes. Online IDEs do not allow file operations.
- Codes are not saved in cloud/remote computer. You can copy and paste the co
Summary: Avoid online IDE
iii. Android
???
Hello, check, check !
The typical first program to check whether compiler, IDE are working or not...
#include<stdio.h>
int main()
{
printf("Hello World);
return 0;
}
fun fact, it is a 7 line code, but same work can be done with only 2 lines of code!
#include<stdio.h>
int main(void){printf("Hello World");}
Write this code character by character in your favorite code editor and run. If everything is alright then you will see your computer welcoming you to the world of programming😉.
Learning Resources
A gentle reminder...
This book is prepared as a supplement to your mainstream learning resource (like Youtube Playlist or any Bangladeshi Book). After Completing topics from your main learning source, you can find additional knowledge and practice problems here. Do not take this book as your main book to learn as we have skipped many things believing that you will learn them in your class or youtube lecture.
Now let's get you started on your learning journey. Choose any of the following ones to start. If you love to read and you can understand well from reading then you may choose option 1. Otherwise choose the other options. (Those are videos. I'll suggest that you try both and then decide.)
Resource | Medium | Link | Comment |
---|---|---|---|
Harvard CS50 | English (Youtube Playlist) | Playlist | Firstly, watch Harvard CS50 to build up concepts. It is Highly Recommended for Basics. |
স্ট্রাকচারড সি/সি-প্লাস-প্লাস প্রোগ্রামিং (ড. মোহাম্মদ কায়কোবাদ , ড. মো. মোস্তফা আকবর , ড. মু. আ. হাকিম নিউটন) | Bangla (Book) | Rokomari Link | Another awesome Bangla Book for starting C Programming for beginners. |
Tamim Shahriar Subeen | Free Bangla (Book) | CPBook part 1 | This is a good book for enthusiasts, but I personally think not much beginner friendly from Bangladeshi perspective. Also, the first edition of the first part of the book is available online for free. You have to buy 2nd & 3rd part. |
Anisul Islam's Documentation | English Text | c-programming-documentation | |
Anisul Islam Youtube Playlist | Bangla (Video) | C Programming | |
College Wallah Youtube Playlist | Hindi (Youtube Playlist) | Playlist | This one is another great playlist for beginners/ |
Bro Code | English (Youtube Playlist) | Playlist | BroCode is an amazing channel for learning !!! If you want to skip BroCode's videos for C, remember this channel name, his videos on Java, HTML, CSS, JavaScript are amazing !!!!! |
C++ : The Cherno | English (Youtube Playlist) | Playlist | Cpp is easy once you have completed C. This channel is highly recommended. |
Programming in Ansi C, 8th Edition | English (Book) | Rokomari Link | This book is widely used. |
How to Start C (Resources)
There are many resources !!!! I am panicked !!! How/Where to start ?
Don't skip CS50, this is a must !!! Select any one from above list as your mainstream learning. But your learning will not complete with one source. YOU HAVE TO EXPLORE OTHER RESOURCES too !!!!!!!!!!
More Resources:
- MIT OpenCourseWare
- https://nptel.ac.in
If you want to download a whole youtube playlist with subtitle, you can check this link.
Primary Knowledge
qwertyuiop
Helpful Tools
CPH extension
link: https://marketplace.visualstudio.com/items?itemName=DivyanshuAgrawal.competitive-programming-helper
Pros:
Online C visual debugger https://pythontutor.com/c.html
Pros:
- visualize variables and changes step by step
Cons/Limitations:
- Can not take input (You have to define values of variables within source code)
- Can not process long code (Suitable for অল্প পরিমাণ কোড and beginners)
Special Characters
Special characters like form-feed (and others) are non-printing characters that perform certain control functions in text processing. Here's a brief explanation of some of them, including form-feed:
1. Form-feed (\f
)
- The form-feed character (
\f
) is used to signal the end of a page in printed documents. In the past, it would move the printer to the top of the next page. - It's rarely used today but might still appear in some contexts (e.g., older printers, plain text files designed for page breaks).
Example:
text = "Page 1 content\fPage 2 content"
print(text)
In this case, \f
represents a form-feed, which would traditionally move content to a new page.
2. Newline (\n
)
- The newline character (
\n
) moves the cursor to the next line. - It is widely used in modern text processing to mark line breaks.
Example:
text = "First line\nSecond line"
print(text)
3. Carriage Return (\r
)
- The carriage return character (
\r
) moves the cursor to the beginning of the same line. - In older systems (e.g., typewriters and early computers),
\r
would reset the cursor position to the start of the line without moving down.
Example:
text = "Hello\rWorld"
print(text)
This would print "World", as \r
causes the cursor to return to the start of the line, overwriting "Hello".
4. Tab (\t
)
- The tab character (
\t
) inserts horizontal spaces, usually equivalent to 4 or 8 spaces, depending on the environment.
Example:
text = "Column 1\tColumn 2\tColumn 3"
print(text)
5. Backspace (\b
)
- The backspace character (
\b
) moves the cursor one position backward, which can effectively delete the previous character when printed.
Example:
text = "Hello\b World"
print(text)
This would print "Hell World" because the backspace removes the "o".
6. Null Character(\0
)
Summary of Common Special Characters:
\f
– Form feed (new page).\n
– Newline (new line).\r
– Carriage return (return to the beginning of the line).\t
– Tab (horizontal space).\b
– Backspace (deletes the previous character).
Each of these special characters can be used for controlling text formatting in various contexts. Today, most of them are used primarily in text processing and formatting, especially in file or console output.
Data Types, Variables and Input Output
Learn
Although there are many data types in c, for now you only need to learn a few. These are int, float, double, char, long long int. You can learn more about them from the following resources.
Link | Note |
---|---|
GeeksForGeeks | Skip void. |
Anisul Islam | Video number 13 to 32 |
Practice
Try to solve the following problems. If you face difficulties or this is your first time solving problem on an online platform please refer here at first. Then if you can't solve it after trying for some time you can see the solution. But try first. Otherwise it won't be beneficial for you.
Problem Link | Hint/Note | Solution |
---|---|---|
Basic Data Types | The description has c++ codes. Don't worry. The Input section stated that Only one line containing the following space-separated values: int, long long, char, float and double respectively. This indicates you need to have 5 variables of type int, long long, char, float and double. You need to read them. And then print them accordingly. To print them in new line you need to use "\n" escape sequence. And remember to not print any extra space. Due to precision given in the sample output you need to print the floating point number using %0.2f and the double using %0.1f. | |
a | v | c |
- | - | - |
a | v | c |
Pre Processor
#define
typedef
Operator & Expression
Theory
Execute this line and think, what is happening...
#include <stdio.h>
int main(void) {
int a, b, c, d, e;
e = 7;
printf("e = %d\n", e);
c = d = e;
printf("c = %d, d = %d, e = %d\n", c, d, e);
a = b = c = d = e = -3; // last "value" or "value of variable" (-3) is going to be assigned to all variables before the last one
printf("%d %d %d %d %d ", a, b, c, d, e);
}
similarly,
#include <stdio.h>
int main(void) {
int a = 1, b = 2, c = -3, d = 0, e = b, f = e;
printf("%d %d %d %d %d %d", a, b, c, d, e, f);
}
You can assign multiple statements in one line with comma. Luckily, you wont have to write multiple lines with semicolon.
#include <stdio.h>
int main(void) {
printf("Hello "), printf("World"), putchar('\n'), printf("input any int value:");
int a = 0;
scanf("%d", &a), printf("\na is %d...", a);
}
this trick is helpful for situation like:
// Note that, this is a cuda code, do not get confused it with a C Programming language code
// the purpose of presenting it is to let you know that you can use this trick in scenarios like this...
#include <cstdio>
#include <cstdlib>
#include <cuda.h>
#define SIZE 1024
__global__ void VectorAdd(int *a, int *b, int *c, int n) {
int i = threadIdx.x;
if (i < n) {
c[i] = a[i] + b[i];
}
}
/*
".cu": "cd $dir && nvcc $fileName -o $fileNameWithoutExt -arch=sm_86 &&
$dir$fileNameWithoutExt",
*/
int main(void) {
int *a, *b, *c;
cudaMallocManaged(&a, SIZE * sizeof(int)), cudaMallocManaged(&b, SIZE * sizeof(int)), cudaMallocManaged(&c, SIZE * sizeof(int));
for (int i = 0; i < SIZE; i++) {
a[i] = b[i] = i;
c[i] = 0;
}
int threadsPerBlock = 256;
int blocksPerGrid = (SIZE + threadsPerBlock - 1) / threadsPerBlock;
// VectorAdd<<<1, SIZE>>>(a, b, c, SIZE); // <<<1, SIZE>>> WAS AN ERROR
VectorAdd<<<blocksPerGrid, threadsPerBlock>>>(a, b, c, SIZE);
// Kernel execution happens here
cudaDeviceSynchronize(); // Wait for kernel to finish
for (int i = 0; i < 10; i++) {
printf("c[%d] = %d\n", i, c[i]);
}
cudaFree(a), cudaFree(b), cudaFree(c);
} // WORKING
Problems
- Click on the title of the problem to submit your solution before looking at our solution.
- We discourage looking at our solution before attempting to solve it by yourself first.
-
Given two numbers X and Y. Print the summation and multiplication and subtraction of these 2 numbers.
Input
Only one line containing two separated numbers \(X, Y (1 ≤ X, Y ≤ 10 ^ 5)\)
Output
Print 3 lines that contain the following in the same order:
- "\(X + Y =\) summation result" without quotes.
- "\(X * Y =\) multiplication result" without quotes.
- "\(X - Y =\) subtraction result" without quotes.
Examples
Input
5 10
Output
5 + 10 = 15 5 * 10 = 50 5 - 10 = -5
Notes
Be careful with spaces.
Hint:
Press the eye icon to reveal hint. Use long long int instead of int Don't forget to use the designated format specifier for long long int (%lld)
Solution
// Press the eye icon to reveal solution. #include <stdio.h> int main() { long long x, y; scanf("%lld %lld", &x, &y); printf("%lld + %lld = %lld\n", x, y, x + y); printf("%lld * %lld = %lld\n", x, y, x * y); printf("%lld - %lld = %lld\n", x, y, x - y); return 0; }
-
Hint:
Press the eye icon to reveal hint. Use long long int instead of int Don't forget to use the designated format specifier for long long int (%lld)
Solution
// Press the eye icon to reveal solution. #include <stdio.h> int main() { long long a, b, c, d, x; scanf("%lld %lld %lld %lld", &a, &b, &c, &d); x = (a * b) - (c * d); printf("Difference = %lld\n", x); return 0; }
Precedence of Arithmetic Operators
Decision Making
if-else
if-else if-else
Ternary Operator
switch case
Looping
Practice problems with loop
Array
String
Practice problems on String
Practice problems on String with Library Functions
Scope
Loops again!
A gentle reminder
Structure & Union
typedef struct
Do not get confused
In C and C++, two different operators are used for calling methods: you use .
if you’re calling a method on the object directly and ->
if you’re calling the method on a pointer to the object and need to dereference the pointer first.
In other words, if object is a pointer, object->something()
is similar to (*object).something()
.
Function
Practice problems with loop
Static variable & Static function
Pointers
Pointer arithmetic
Pointer passing to function
Intro to Algorithm
Time and space complexity - basic knowledge
It is better to have low time complexity and space complexity. But in many cases, we have to choose either one to solve our problem. Sacrificing one rather achieving both goal (goal of making minimum TC and SC) may be necessary but don’t worry that much now as a beginner. Focus on your logic building and Approach of solving problems.
Binary Search
Recursion
PRACTICE Recursion
Some of the following problems are directly copied from Zobayer Hasan vai's blog post on recursion. We are grateful to his open contributions towards learning CS.
You can use the Competitive Programming Helper (cph) extension in VS code to make your practice easier though it wont make a big difference.
ALL THE BEST FOR YOUR HARD WORK.
Problems on Recursion
-
Print 1 to 50 with a recursive function.\
Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
Hint:
// recursive call printer(number + 1); // value of number increments here
Solution:
#include <stdio.h> void printer(int number) { // printf("in this recursive call, number is %d \n", number); // Base Case if (number > 50) { // in a new recursive call, if the value of number is greater than 50, recursive call should terminate return; } else { printf("%d ", number); // recursive call printer(number + 1); // value of number increments here } } int main(void) { printer(1); }
-
Print 1 to 50 reversely with a recursive function.\
Output:
50 49 48 47 46 45 44 43 42 41 40 39 38 37 36 35 34 33 32 31 30 29 28 27 26 25 24 23 22 21 20 19 18 17 16 15 14 13 12 11 10 9 8 7 6 5 4 3 2 1
Hint:
uno reverse... write down only 5 recursive call (with code and variable value) in your notebook
Solution:
#include <stdio.h> void printer(int number) { if (number > 50) { return; } else { printer(number + 1); // notice the change here printf("%d ", number); // and think, how ? } } int main(void) { printer(1); }
-
Print out the series $$ 1 + 2 + 3 + ... + n $$ and determine the sum.
Input:5
Output:
1 + 2 + 3 + 4 + 5 = 15
Hint:
#include <stdio.h> int sum_it(int num, int limit) { if (num > limit) { printf("= "); return 0; } else { printf("%d ", num); if (num != limit) { printf("+ "); } return num + sum_it(num + 1, limit); } } int main(void) { int limit = 0; scanf("%d", &limit); int sum = sum_it(1, limit); printf("%d", sum); } // done
-
You will be given an array of integers. Write a recursive solution to print it in (inputted) order.
Input:5 69 87 45 21 47
Output:
69 87 45 21 47
Hint:
-
Same as before. Add index number (starting from 1) to each int output in (inputted) order.
Input:5 69 87 45 21 47
Output:
1.69 2.87 3.45 4.21 5.47
-
Same as before. Add index number (starting from n) to each int and get output in reverse order.
Input:7 69 87 45 21 47 76 2
Output:
7.69 6.87 5.45 4.21 3.47 2.76 1.2
-
You will be given an array of integers. Write a recursive solution to print it in reverse order. Input:
5 62 87 45 24 47
Output:
47 24 45 87 62
-
Same as before. The program will print inputted numbers except even integers. Input:
5 62 87 45 24 47
Output:
47 45 87
-
Use recursion to find max among 2 integers.
-
Use recursion to find the max among 3 integers.
-
Use recursion to find min among 2 integers.
-
Use recursion to find min among 3 integers.
-
Write a recursive function to print an array in the following order.
[0] [n-1] [1] [n-2] ... [(n-1)/2] [n/2]
Input:
5 1 5 7 8 9
Output:
1 9 5 8 7 7
-
Write a recursive program to remove all odd integers from an array. You must not use any extra array or print anything in the function. Just read the input, call the recursive function, and then print the array in main().
Input:6 1 54 88 6 55 7
Output:
54 88 6
-
Write a recursive solution to print the polynomial series for any input n: $$ 1 + x + x^2 + ... + x^{n-1} $$
Input:5
Output:
1 + x + x^2 + x^3 + x^4
-
Write a recursive solution to evaluate the previous polynomial for any given x and n. Like, when x=2 and n=5, we have $$ 1 + x + x^2 + ... + x^{n-1} = 31 $$
Input: 2 5 Output: 31 -
Write a recursive program to compute n!
Input: 5 Output: 120 -
Write a recursive program to compute the
n-thFibonacci number. 1st and 2nd Fibonacci numbers are 1, 1.
Input: 6 Output: 8 -
Write a recursive program to determine whether a given integer is prime or not.
Input: 49 999983 1 Output: not prime prime not prime -
Write a recursive function that finds the gcd of two non-negative integers.
Input: 25 8895 Output: 5 -
Write a recursive solution to compute the LCM of two integers. You must not use the formula lcm(a,b) = (a x b) / gcd(a,b); find lcm from scratch...
Input: 23 488 Output: 11224 -
Suppose you are given an array of integers in an arbitrary order. Write a recursive solution to find the maximum element from the array.
Input: 5 7 4 9 6 2 Output: 9 -
Write a recursive solution to find the second maximum number from a given set of integers.
Input: 5 5 8 7 9 3 Output: 8 -
Implement linear search recursively, i.e. given an array of integers, find a specific value from it. Input format: first n, the number of elements. Then n integers. Then, q, the number of queries, then q integers. Output format: for each of the q integers, print its index (within 0 to n-1) in the array or print 'not found', whichever is appropriate.
Input: 5 2 9 4 7 6 2 5 9 Output: not found 1 -
Implement binary search recursively, i.e. given an array of sorted integers, find a query integer from it. Input format: first n, the number of elements. Then n integers. Then, q, the number of queries, then q integers. Output format: for each of the q integers, print its index (within 0 to n-1) in the array or print 'not found', whichever is appropriate.
Input: 5 1 2 3 4 5 2 3 -5 Output: 2 not found -
Write a recursive solution to get the reverse of a given integer. The function must return an int.
Input: 123405 Output: 504321 -
Read a string from keyboard and print it in reversed order. You must not use any array to store the characters. Write a recursive solutions to solve this problem.
Input: helloo Output: oolleh -
Write a recursive program that determines whether a given sentence is palindromic or not just considering the alpha-numeric characters ('a'-'z'), ('A'-'Z'), ('0'-'9').
Input:madam
Output:
palindromic
Input:
hulala
Output:
not palindromic
-
Implement prime number determining algorithm recursively.
Hint:
isPrime(num, j + 2);
Solution:
int isPrime(int num, int j) { // RECURSIVE if (num <= 1) { return false; } if (num % 2 == 0) { return false; } if (num % j == 0) { return false; } if (j * j <= num) { isPrime(num, j + 2); } return true; }
-
Implement strcat(), stracpy(), strcmp() and strlen() recursively.
Input: test on your own
Output: test on your own -
If you already solved the problem for finding the nth fibonacci number, then you must have a clear vision on how the program flow works. So now, in this problem, print the values of your fibonacci function in pre-order, in-order and post-order traversal. For example, when n = 5, your program calls 3 and 4 from it, from the call of 3, your program calls 1 and 2 again....... here is the picture: Input:
5
Output:
Inorder: 1 3 2 5 2 4 1 3 2 Preorder: 5 3 1 2 4 2 3 1 2 Postorder: 1 2 3 2 1 2 3 4 5
-
Convert a Decimal number to Binary.
Hint:return Decimal_to_Binary_RECURSIVE(i / 2) ... ;
Solution:
#include <stdio.h> int Decimal_to_Binary_RECURSIVE(int i) { if (i == 0){ return 0; } return Decimal_to_Binary_RECURSIVE(i / 2) * 10 + i % 2; } int main(void) { int i = 0; scanf("%d", &i); printf("the binary of the decimal num %d is %d", i, Decimal_to_Binary_RECURSIVE(i)); }
-
All of you have seen the tower of Hanoi. You have 3 pillars 'a', 'b' and 'c', and you need transfer all disks from one pillar to another. Conditions are, only one disk at a time is movable, and you can never place a larger disk over a smaller one. Write a recursive solution to print the moves that simulates the task, a -> b means move the topmost of tower a to tower b.
Input:3
Output:
a -> c a -> b c -> b a -> c b -> a b -> c a -> c x
Dynamic Memory Allocation
More on Memory
Linked List
Prerequisites:
Structure(must), Typedef, Pointers (must), Memory, importance of data type, stack and heap region of memory, dynamic memory allocation(malloc) and how it works.
Practice Problem
- Problem: You will be given an array of integers. Use
malloc()
to allocate memory in the heap. Write a recursive solution to print them in (inputted) order. ??????????????
Input:
5
69 87 45 21 47
Output:
69 87 45 21 47
int main(int argc, char *argv[])
File Operations
Notes on File Operation with C
Function | Description |
---|---|
fopen() | opens new or existing file |
fprintf() | write data into the file ["ফাইল" স্ট্রিম, কি স্ট্রিং ফরম্যাটে সেইভ করব, স্ট্রিম সোর্স] |
fscanf() | reads data from the file while(fscanf(fp,"%d",&temp_num)!=EOF){ |
fputc() | writes a character into the file |
fgetc() | reads a character (in loop, character by character) from file while ((c = fgetc(fp)) != EOF) {printf("%c", c); |
fputs() | put(WRITE) a whole string to the file |
fgets() | using while(fgets!=NULL), reads "line by line" from file with whitespace and sets to buffer string while (fgets(str, 300, f) != NULL) {printf("%s", str); ; while (fgets(line, sizeof(str_line), f)) { |
fclose() | closes the file |
fseek() | sets the file pointer to given position |
fputw() | writes an integer to file |
fgetw() | reads an integer from file |
ftell() | returns current position |
rewind() | sets the file pointer to the beginning of the file |
notes and tricks
scanf("%[^EOF]",str);
read full file at oncefreopen("fileout.txt", "w", stdout);
directly save into the file (steam bypass)fprintf()
e W+ dile full file override hoy, r+ thakle shudhu oi position er char gulofscanf()
: format%c
ar%s
er upor depend kore kivabe source theke ek ek kore collect korbe. jodi%s
use kori then: ek ekta word by word catch kore, so, _buff (steam) e ekta ekta kore word dhukse, without counting whitespace characters, so, while loop er vitore amakeprintf()
er vitore ekta space ditei hosse Jemon silo temon paite holefscanf
er vitoreC
arprintf
er vitoreS
.int temp = 0; while (fscanf(f_input, "%d", &temp) != EOF) {
while (fscanf(ptr, "%c", &str[i]) != EOF && i < 199) { i++;}
while((ptr=strstr(str,word))!=NULL) {memmove(ptr,ptr+len,strlen(ptr+len)+1);}
Practice Problems
These problems are directly taken from https://github.com/joydeb1729/File_Practice_C. For solutions, please visit this github repo. You will find solutions in respective folder.
@main_author : Durjay1729
Github: https://github.com/joydeb1729/
1. Write a C program to read numbers from a file and write even, odd and prime numbers to three separate file.
input->
73771782 81296771 79982326 75332246 10128193
81643413 76259734 94432076 50063976 91748657
42311916 -1920042 90747362 53851612 43498487
73193311 96685173 39019033 8630045 59322952
Output->
even.txt
73771782
79982326
75332246
76259734
94432076
50063976
42311916
-1920042
90747362
53851612
59322952
59322952
odd.txt
81296771
81643413
91748657
43498487
96685173
8630045
prime.txt
10128193
73193311
39019033
2. Write a C program to compare two files.
input -> File1.txt
Learn C programming at KU.
Working with files and directories.
File2.txt ->
Learn C programming at KU.
Working with chatgpt and copilot.
Output->
Files are not equal.
Line: 2, column: 14
3. Write a C program to copy contents from one file to another file.
Hint with Solution:
#include <stdio.h>
#include <stdlib.h>
int main(void) {
FILE *f1 = fopen("file3_1.txt", "r"), *f2 = fopen("file3_2.txt", "w");
if (f1 == NULL || f2 == NULL) {
printf("ERROR OPENING FILE");
exit(EXIT_FAILURE);
}
char c1;
while (1) {
c1 = fgetc(f1);
fputc(c1, f2); // focus
if (c1 == EOF) { // EOF has been written to f2 and now loop needs to be stopped
break;
}
}
fclose(f1), fclose(f2);
} // DONE
4. Write a C program to count characters, words and lines in a text file.
input->
I love programming.
Working with files in C programming is fun.
I am learning C programming at KU.
Output->
Total characters = 106
Total words = 18
Total lines = 3
5. Write a C program to remove a word from text file.
file.txt
I love programming.
Programming with MM Sir is fun.
Learning C programming at KU is simple and easy.
Take input from user.
6. Write a C program to remove specific line from a text file.
file.txt
I love programming.
Programming with MM Sir fun.
Learning C programming at KU is simple and easy.
take input from User
7. Write a C program to remove empty lines from a text file.
file.txt
I love programming.
Programming with files fun.
Learning C programming at Codeforwin simple and easy.
8. Write a C program to find and replace a word in a text file.
file.txt
I love programming.
Programming with files is fun.
Learning C programming at KU is simple and easy.
replace "file" with "chatgpt"
9. Write a C program to count occurrences of all words in a text file.
file.txt
I love programming.
Programming with files is so fun.
Learning C programming at KU is so simple and easy.
take input from User
10. Write a C program to convert uppercase to lowercase character and vice versa in a text file.
file.txt
I love programming.
---------PROGRAMMING IN C---------
Learning C programming at KU is simple and easy.
output->
i LOVE PROGRAMMING.
---------programming in c---------
lEARNING c PROGRAMMING AT ku IS SIMPLE AND EASY.
NOTE: the following four questions are previous lab test questions set by Dr Manishankar Mondal Sir on Strings. We have modified them to introduce file handling concepts.
11. Write a program that takes a file containing string with spaces from the user. Your program will then determine the following and save them in txt files.
- Find the individual words and store them in a two-dimensional array. Save the words.
- Count the number of vowels in each word. Save the counts for all words in a file.
- Print the appearance counts of the distinct words? Draw a histogram considering the appearance counts and save them in another file.
Sample Input file input.txt
:
I know the man. the man is a good human being. the man has good characteristics. man man man man the the the the good good
First Output file:
I, vowel count = 1
know, vowel count = 1
the, vowel count = 1
man, vowel count = 1
the, vowel count = 1
man, vowel count = 1
is, vowel count = 1
a, vowel count = 1
good, vowel count = 2
human, vowel count = 2
being, vowel count = 2
the, vowel count = 1
man, vowel count = 1
has, vowel count = 1
good, vowel count = 2
characteristics, vowel count = 5
man, vowel count = 1
man, vowel count = 1
man, vowel count = 1
man, vowel count = 1
the, vowel count = 1
the, vowel count = 1
the, vowel count = 1
the, vowel count = 1
good, vowel count = 2
good, vowel count = 2
Second output file distinct_words_appearance_counts.txt
:
I: *
know: *
the: ***
man: ***
is: *
a: *
good: ****
human: *
being: *
has: *
characteristics: *
12. Assume that you have an array of 20 positive integers in a file. Write a program to RECURSIVELY determine the SUMMATION of all prime integers in the array. You SHOULD write the prime testing function recursively. The array will be taken as input from file and append the answer to the input file at last.
input.txt
4
345
45
78
324
5
45
453
435
37
78
423
453
34
578
31
374
19
11
111
output:
4
345
45
78
324
5
45
453
435
37
78
423
453
34
578
31
374
19
11
111
sum:103
13. Write a program to take a string with spaces from a file. Your program will save the string to output.txt
after trimming it. Let us assume that the input string is " I live in a beautiful village. ". Your program will remove the spaces from the front and back of the string and then save it. Thus, the output will be "I live in a beautiful village." For trimming (i.e., for removing spaces from the front and back) you need to create a user-defined function called "trimstring" that takes a string as a parameter.
14. "LTrim", "RTrim", and "MTrim", and "CountOccurrence"
Write a program that will take a string with spaces from file as input. Write four functions named "LTrim", "RTrim", and "MTrim", and "CountOccurrence". Each of these functions will take a string as a parameter.
"LTrim" will delete all the spaces from the left hand side of the parameter string and will return the string that will be obtained after deleting the left side spaces.
"RTrim" will delete all the spaces from the right hand side of the parameter string and will return the string that will be obtained after deleting the right side spaces.
"MTrim" will keep only one space between every two consecutive words. There can be multiple spaces between two words in a string. Only one space will be kept and other spaces will be deleted.
And, "CountOccurrence" will recursively count the occurrences of "abc" in a parameter string and will return the count. This function might also take other parameters as you wish to accomplish the task.
Contributions
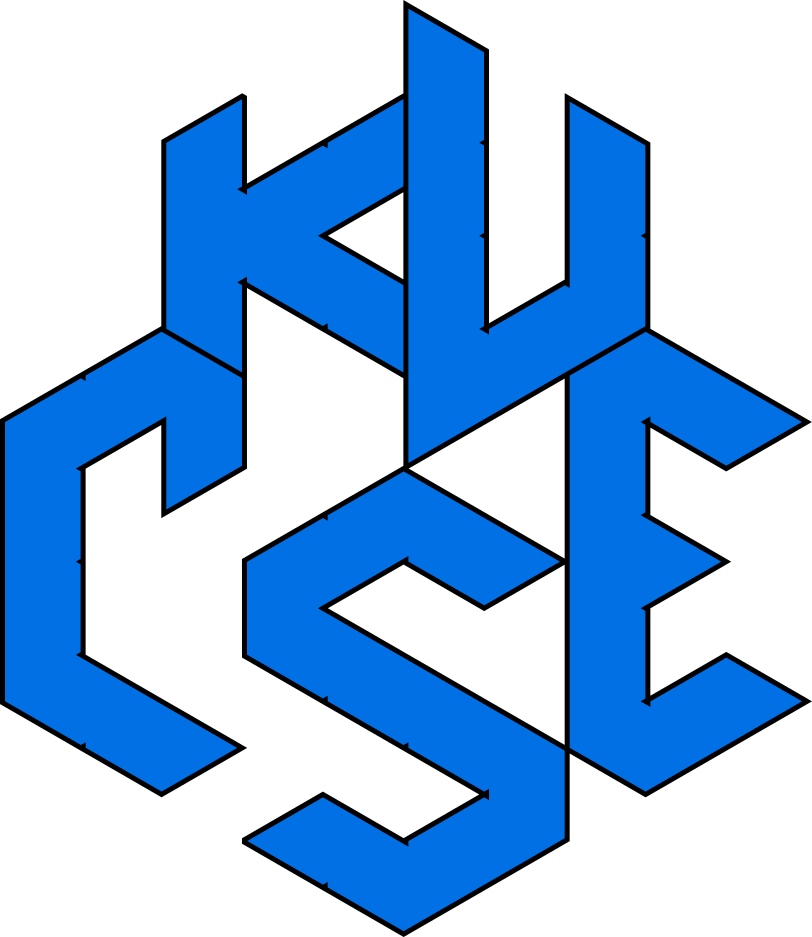
Back Story, Our Motivations
During our 1st year 2nd term, me along with my close 3 friends felt necessity of collaboration and assistance to start programming life for beginners. We decided to help our
After our term final examination, during Eid Vacation, my friend, Ibnul Abrar Shahriar Seam sent me a link https://structured-programming-ku-cse.blogspot.com/ and added me as a contributor. Later, my classmates Azmain Inquaid Haque, and Joydeb Gan Prokas (Durjay) directly contributed to this book.
Later, a lot happened, we achieved the 2nd independence of Bangladesh on "36th July 2024" (05 August 2024) with the heroic patriotism of Abu Sayed Vai, Mir Mugdha Vai, and many more which motivated us to share our knowledge and thoughts to build this nation. To make it freely available for everyone and everyone's contribution, we decided to make it open by hosting in Github with mdBook.
We look forward to rebuild our motherland as well as making this world better.
...